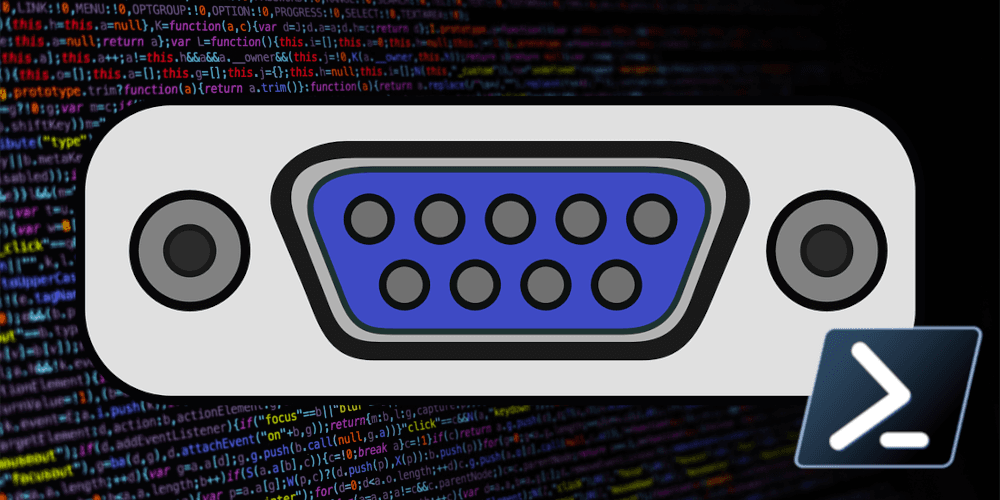
SerialShell
Photo Credits: Unsplash, Tech Icons, and Pixabay
Project Link: https://github.com/niwamo/SerialShell
Introduction
Windows does not have a native tool for console sessions over serial connections. Historically, that gap has been filled with putty
, which I have long considered obnoxious.
(There's nothing wrong with it, but I dislike both the need to download an executable and the need to use a GUI for a CLI session - especially when native tools are perfectly capable of providing the same functionality.)
It's generally known that PowerShell (via .NET assemblies) is capable of providing this functionality, and there are a large number of StackOverflow posts and code samples with rudimentary serial session tools for PowerShell. These generally involve a while loop and a Read-Host
command. While this works in some scenarios, it prevents interactivity - Read-Host
is a blocking function and waits for an "ENTER" key-press before returning.
Benefits of SerialShell
- Pure PowerShell serial sessions - no
putty
necessary - Fully-interactive serial sessions; Tab-complete, command history searching, and interactive terminal apps all work perfectly fine
Approach
Full interactivity is achieved by:
- Removing the blocking user input function (e.g.,
Read-Host
) - Sending all key-presses to the remote host as soon as they are detected (using
[Console]::KeyAvailable
and[Console]::ReadKey($true)
) - Using a Background Job/Event Subscriber to monitor for and handle data received from the remote host
Additionally, key-presses that do not directly translate into serializable text are translated into their respective ANSI escape codes before transmission. If you're interested in understanding the need for this, check how the output of the arrow keys, HOME, and END differ from alphanumeric keys with the following script:
while ($true) {
$k = [console]::readkey($true)
if ( $k.keychar -eq "z" ) {
break
}
Write-Host "$([byte]$k.keychar), $($k.key)"
}
(See my article on ANSI escape codes here)
Dependencies
Full interactivity is made possible by Windows' support for ANSI escape codes, which are sent by the remote host to (for example) re-draw the entire console screen during a vim
session. Support was added in Windows 10 v1511.
Testing
This has been tested with a Windows 11 client connecting over UART to a UDM SE Pro.
Usage
New-SerialSession -COMPort 5 -BaudRate 115200 -Parity None -DataBits 8 -StopBits one
Note: COMPort
and BaudRate
are the only mandatory parameters.